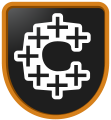
General C++
The VMK in this section cover many topics related to C++ programming. If you want to be serious about programming in C++, you should fully understand each of the topics covered here.
1: Number Systems
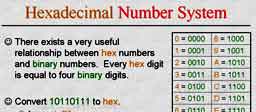
In this VMK I show you how number systems work. I describe the decimal, binary and hexadecimal number systems in detail. At the end, I show you how to use Visual C++ to view decimal (integers) and hexadecimal numbers.
2: Computer Logic
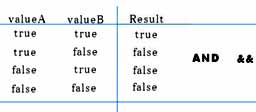
I describe how to write a program that can make decisions. I demonstrate the use of the if-else structure and then the AND, OR, NOT, bitwise OR and bitwise AND are described and used.
3: Switch and Enum Keywords
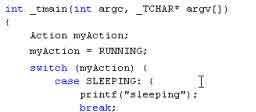
You can make your code more readable by replacing if-else statements with switches working from enumerated variables. This VMK shows you how to do just that.
4: Looping Structures
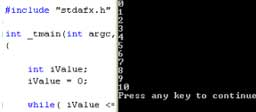
I show you how to use the while and the for loop in this VMK so that you can perform repeated tasks in your programs. Near the end I also describe how random numbers work in C++.
5: Data Types
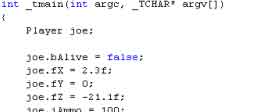
A number of commonly used C++ data types are described in this VMK. I discuss the int, float, double, and char types. After that, I describe how to use arrays and how they work a little differently with the char data type to make strings. We group data types into struct's and lastly I show you how to use a union.
6: Dynamic Memory and Variable Scope
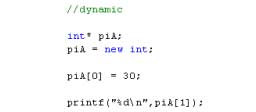
I describe the important concept of variable scope in this VMK. I also show you how to use, create and delete dynamic memory in C++.
7: Using Functions
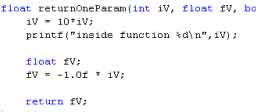
This VMK is all about functions and how to use them. I demonstrate how to pass variables into functions and how to get values back. I explain the difference between passing variables by value, and passing variables by reference. I show you how to use a function to return multiple values to you and I describe how you can use functions with pointers and array's of variables.
8: Libraries and Projects
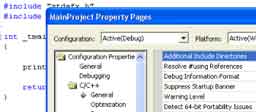
I show you how to setup a visual studio solution to contain multiple projects. One project contains the main() entry point and the other is a static library which we link to. I also show how build order dependence plays a role when using multiple projects in a solution.
9a: Struct vs Class
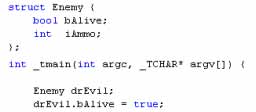
The differences between a struct and a class are described in this VMK. I also go onto show how to use public and private functions and variables within a class and how to use a class constructor.
9b: Static Variables and Functions
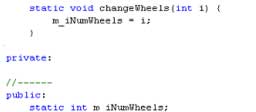
I demonstrate how to use static variables and static functions inside of a class.
9c: Class Inheritance
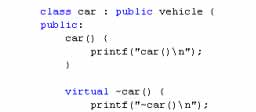
I show you how to use a very powerful object oriented technique called inheritance in this VMK. Public, private and protected functions and variables are used and virtual functions are explained.
9d: Copy Constructor
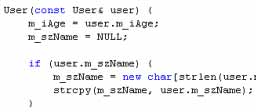
When using dynamic memory inside of classes you will need to create a copy constructor to copy one class's values to another. In this VMK I show you why you need copy constructors and how to make them.
10: Standard Template Library
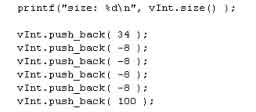
The first part shows you how to use std::vector and some of it's common functions. This second part shows you how to use std::string and std::map.
11: File Handling
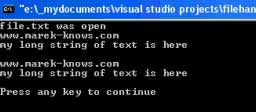
In this VMK I show you how to work with ascii (text) files to read data in and write data out to a file. I show you how to setup Visual Studio and how to read in a bunch of data into one variable, or parse input into individual variables like integers, strings or floating point numbers.
12: Install VC 2005 Express with PSDK
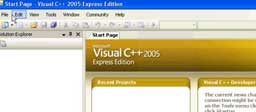
I show you how to download and install the free Visual C++ 2005 Express compiler from Microsoft. I also show you how to download and install the Platform SDK required if you want to use this compiler to follow along with the game development VMK's or if you want to do any windows type programming.
13: Visual C++ Debugger
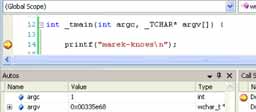
In this VMK you will learn how to use the Visual C++ Debugger. I show you how to create breakpoints (always break and conditional). I show you how to watch your variables and how to change them while your program is running. We also trace through the program flow by using the calling stack. At the end I also show you how you can comment your code so that software development is a little easier.
14: Templates
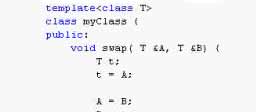
In this video I explain the benefits of using templates in C++. I demonstrate how to template a function and also how to template a class.
15: Threads
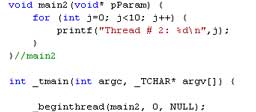
I start by showing how to create a 2nd thread and how to pass data to and from it. A Mutex is used to monitor when a thread is finished and a Critical Section is used to protect data shared between threads. Finally I show how to use a thread inside a class.
16: Random Numbers
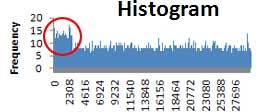
In this video I describe how random numbers work in C++. How you can create a range of random numbers, and also what things you should look out for if you truly want a set of random numbers.
17: zLib Compression
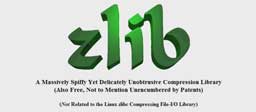
Data compression is discussed in this VMK. By the end of the video you will see how to compress and uncompress data using the zlib library.
18: Coding Style
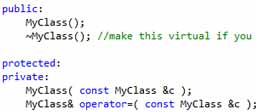
In this video I speak about the C++ coding style that I'm going to try to stick to from this day forward.
19: 3rd Party AddOn Tools
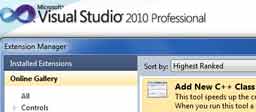
If you are using Visual Studio 2010 Professional, you can install AddIn tools to extend the functionality of your development environment. In this VMK I demonstrate the tools that I use when programming in C++.
20: Unique Pointers
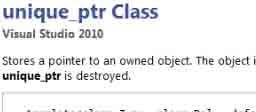
Unique pointers are very handy in VC++ 2010. They let you manage your dynamic memory in a smart way making sure that memory is deallocated when your pointers go out of scope.
21: Explicit Constructors
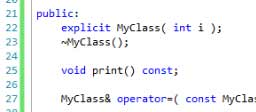
In this video I explain when you should be using explicit constructors. I demonstrate what will happen if you do not use them, and I also show when you should not use explicit constructors.
22: i++ vs ++i
I show the differences between using postfix (i++) operators as opposed to using prefix (++i) operators. It is especially important to choose the correct operator when working with looping structures like for loops.
23: Bit Fields
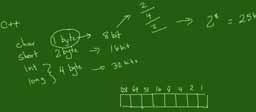
In this video I describe how to use bit fields in C++. I demonstrate how the compiler packs bits together and I point out the pit falls that people can fall into when using bit fields.
24: PNG Loader
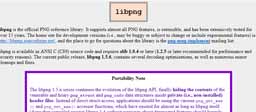
In this video I show how to download, install, and use the libpng library to work with images encoded in the png file format. By the end of this video you should be able to load and parse any png file in your own projects.
25: Exceptions
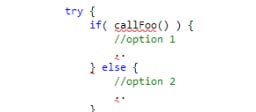
In this VMK I introduce how to use C++ exceptions. We explore how to write try and catch statements, how to throw values and classes, and I demonstrate the differences between throwing from a function and throwing from a class.
26: Console Colors
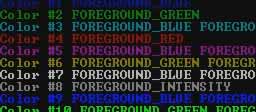
Using the Windows API, I demonstrate how to change the foreground and background color of text, how to control the placement of text in the window and how to change the title.
27: Virtual Destructor
This video shows you why virtual destructors are important and when you need to use them when writing code.