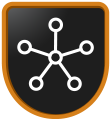
Network Programming
This series will teach you to use windows sockets to create internet, intranet, and other network-capable applications to transmit data across the wire, independent of the network protocol being used. We will be creating TCP and UDP networks for both client and server applications using C++.

Looking for server...
0: Introduction
Welcome to network programming in Visual Studio 2010 using C++. This VMK describes what will be covered in the series.
1: Setup
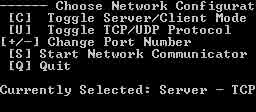
I show how to setup the Network Manager project using Microsoft Visual Studio 2010. I also create a menu system that allows the user to choose whether they want to create a Server or a Client application, whether it will use TCP or UDP, and select which port number to use.
2: TCP Server
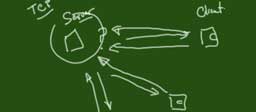
In this VMK I create the network manager class which is used throughout the series. In this video I show how to write the code to create a TCP server.
3: TCP Client
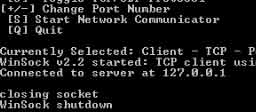
The TCP client code is written in this vmk. Using the client, you will now be able to connect to the tcp server developed in the previous vmk.
4: Send String
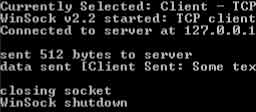
In this video I create a function that lets us send a fixed length string using the TCP connection that we established between the client and the server.
5: Receive String
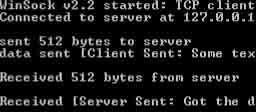
I clean up the main function to show more clearly what the Server and Client applications do. Then I add the function to the NetworkManager that lets us receive string data sent over the network. Finally I test the functionality so that it works in both directions, Server to Client and Client to Server.
6: UDP Send & Receive
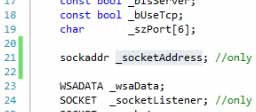
The sendString and receiveString function calls are updated in this VMK to allow UDP communication to send and receive string data.
7: UDP Server
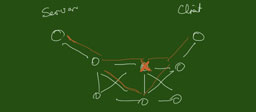
In this video I show how to setup the server portion of the network if you plan on using UDP communication.
8: UDP Client
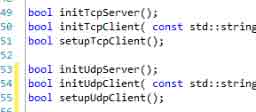
In this video I wrap up the missing component that is needed to do UDP communication across the network. We finally see how to initialize the _socketAddress member variable.
9: Using Real IP Addresses
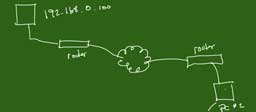
In this video I replace the loopback IP address (127.0.0.1) with the real IP address used on my local area network. I also explain how to prepare remote computers so that they will be able to run the NetworkManager code.
10: Memory Clean Up
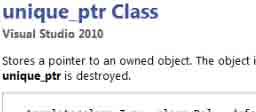
In this video I cleanup the code used to allocate and deallocate dynamic memory in the network manager using the unique_ptr class. After this smart pointer is introduced, we won't have to call delete explicitly.
11: Non-Blocking WinSock
In this video I present the different ways in which a WinSock program can be written so that it does not block your main execution thread.
12: Non-Blocking TCP Server
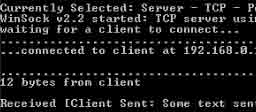
I demonstrate how to make the winsock code not block the TCP server. After switching to non-blocking mode, we explore the error messages that we get with our existing code and then we go through to fix them all to make the code run once again.
13: Non-Blocking TCP Client
In this VMK I update the TCP client code so that it too will run using non-blocking sockets.
14: Non-Blocking UDP Communication
In this video I show how to modify the udp server and udp client code so that they both will run using non-blocking sockets.
15: Send String Packet
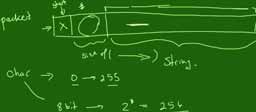
In this video I introduce the concept of a packet. I show how to form a sample packet that can be used to transmit strings containing different lengths of characters.
16: Receive String Packet
In this video tutorial, I show how to decode the string packet that was sent in the previous VMK so that we can see what is being transmitted across the network.
17: GameData Class
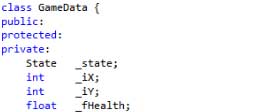
In this video I create a generic GameData class which holds different data types. In the next VMK we'll see how to transmit the contents of the class across the network.
18: GameData Packet
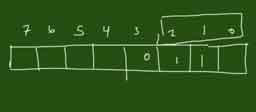
The GameData class created in the previous VMK is converted to a packet in this VMK so that the data contained in the class can be transmitted across the network.
19: Float Conversion Tests
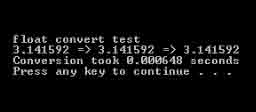
In the previous VMK I left out how to transmit floating point numbers across the network. In this VMK I demonstrate different ways in which this can be done.
20: Bitfield GameData Packet
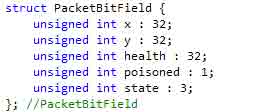
The data stored in the GameData class is packaged into a bitfield packet in this VMK to make it easier to transmit and receive data across the network.
21: Chatter Demo
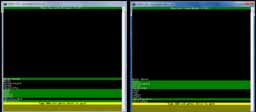
In this video I demonstrate a simple chat program that can now be built with all the tools we developed so far.
Unfortunately due to lack of interest in this VMK series from users, I never created anymore videos in this series to show how to actually create this chatter application.
Unfortunately due to lack of interest in this VMK series from users, I never created anymore videos in this series to show how to actually create this chatter application.